Receive Asaas Events at Your Webhook Endpoint
Set up a webhook URL to keep your application always up-to-date with API integration
Why Use Webhooks?
If you aim to keep payment and customer data synchronized with your application when creating an integration, webhooks are the best solution. They act as a "reverse API," where Asaas makes an HTTP REST call to your application.
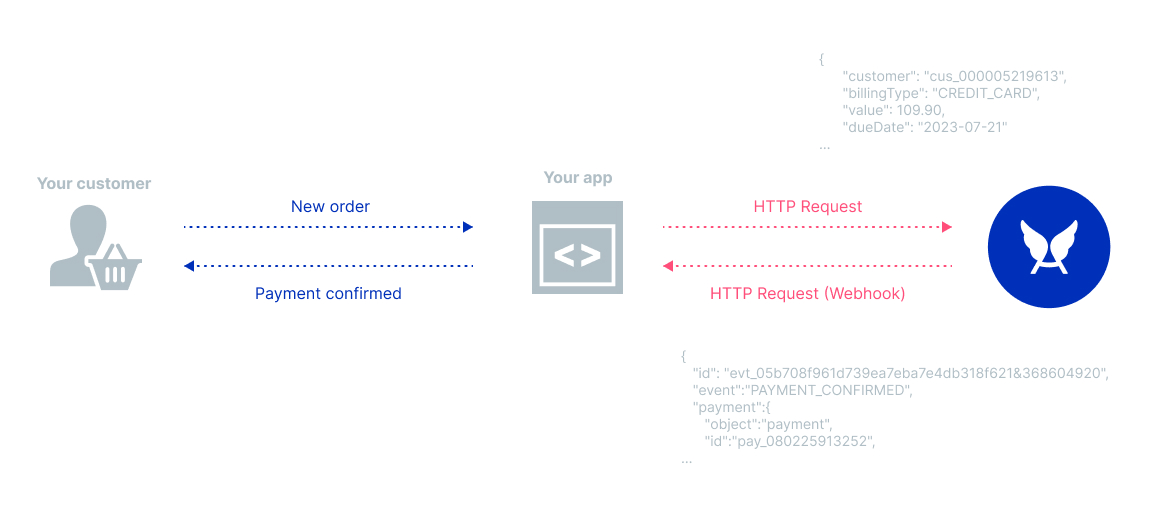
To enable webhook event reception, you need to configure the URL that will receive the events, which can be done via the interface by accessing the web application, or via API. You can register up to 10 different webhook URLs, and in each one, you define which events you want to receive.
The Event Object
Events are JSON objects sent via Asaas webhooks. They notify when an event has occurred in your account.
Through it, you will have access to the id
, event
indicating which event occurred, and the object of the entity to which the event belongs. In the example below, we have the payment
object with the data of the respective charge.
{
"id": "evt_05b708f961d739ea7eba7e4db318f621&368604920",
"event":"PAYMENT_RECEIVED",
"payment":{
"object":"payment",
"id":"pay_080225913252",
...
}
}
Webhooks are the way you subscribe to events and receive notifications in your application whenever the event occurs.
Event Types
Events are divided into categories related to the entity to which they belong. Check out the Webhook Events page to see each one.
Get Started Here
To start receiving events via webhooks in your application, follow the steps below:
- Access the Sandbox environment;
- Create an endpoint in your application to receive HTTP POST requests;
- Configure your webhook using our web application or via API;
- Test your webhook;
- Debug any issues with events;
- Once tested and validated, replicate the settings in the Production environment;
- Keep your webhook secure.
Create an Endpoint
Create an HTTP endpoint (for testing on localhost) and HTTPS (for production use) that expects to receive an event object in a POST event. This endpoint should also return a 200 response as quickly as possible to avoid issues with the event synchronization queue.
Below is a basic example using Node.js:
const express = require('express');
const app = express();
app.post('/payments-webhook', express.json({type: 'application/json'}), (request, response) => {
const body = request.body;
switch (body.event) {
case 'PAYMENT_CREATED':
const payment = body.payment;
createPayment(payment);
break;
case 'PAYMENT_RECEIVED':
const payment = body.payment;
receivePayment(payment)
break;
// ... handle other events
default:
console.log(`This event is not accepted: ${body.event}`);
}
// Return a response to indicate the webhook was received
response.json({received: true});
});
app.listen(8000, () => console.log('Running on port 8000'));
Configure Your Webhook
You can configure a new webhook via the web application or via API.
We recommend that, to test your webhook and integration, you first create a Sandbox account. Check out our documentation on Sandbox and follow the steps. You can also follow the tutorials on creating a webhook:
Test Your Webhook
With the webhook configured in Sandbox, you can test your code that is on localhost using some applications that expose your local code on the web.
We recommend using a trusted application like ngrok or Cloudflare Tunnel. With both applications, you can define a URL that you can use in your webhook configuration.
Debug Integration with Webhooks
You can easily debug your webhook through our Webhook Logs page. Access User Menu > Integrations > Webhook Logs.
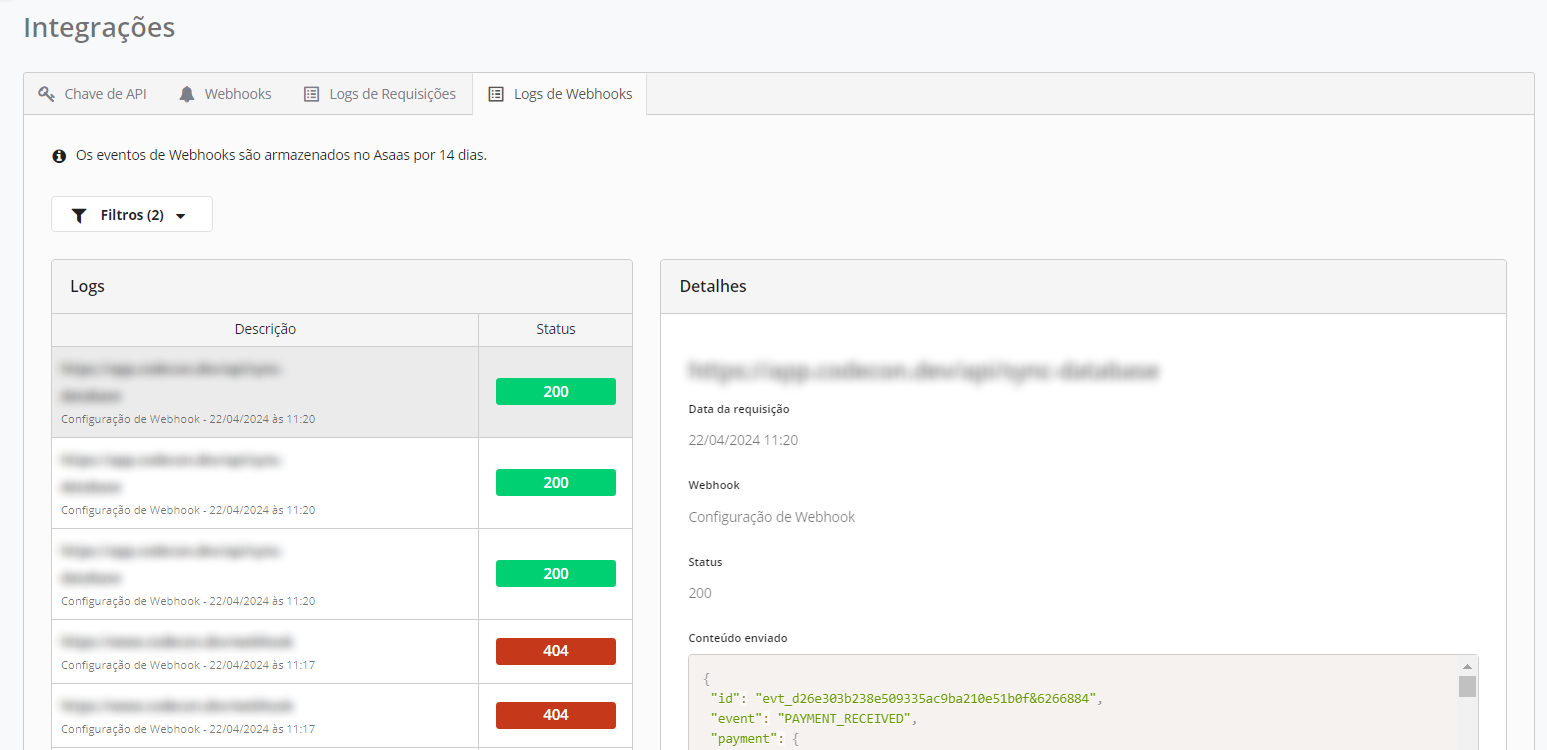
On this page, you can view all requests sent via webhook to your application, the status returned by your server, and also the content sent. This page is also relevant when you encounter issues with the paused synchronization queue, check the documentation for more details.
Keep Your Webhook Secure
It is highly recommended that you keep your integration and all your webhooks secure. As a recommendation, Asaas suggests:
- Trust only Asaas IPs for webhook calls: you can block via firewall all IPs making calls to your webhook URLs, except the official Asaas IPs.
- Configure an
authToken
: when creating a new webhook, you can set a unique code for it. Create a strong hash, preferably a UUID v4, and always check theasaas-access-token
header to ensure that this is a legitimate call.
Updated 12 months ago